Source Plugin
Source Plugins
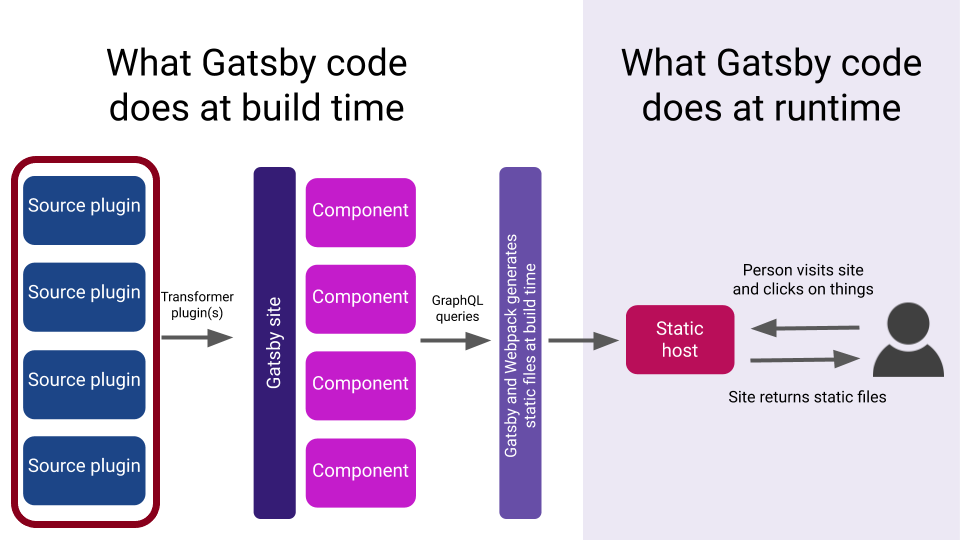
bash
npm i gatsby-source-filesystem
bash
npm i gatsby-source-filesystem
gatsby-config.js
js
module.exports = {
plugins: [
'gatsby-plugin-sass',
{
resolve: 'gatsby-source-filesystem',
options: {
name: 'src',
path: `${__dirname}/src`,
},
},
],
};
gatsby-config.js
js
module.exports = {
plugins: [
'gatsby-plugin-sass',
{
resolve: 'gatsby-source-filesystem',
options: {
name: 'src',
path: `${__dirname}/src`,
},
},
],
};
Build a Page with Data from Source Plugin
src/pages/all-code.js
jsx
import { graphql } from 'gatsby';
import * as React from 'react';
import { Layout } from '../components/layout';
const AllCodePage = ({ data }) => {
return (
<Layout>
<table>
<thead>
<tr>
<th>relativePath</th>
<th>prettySize</th>
<th>extension</th>
<th>birthTime</th>
</tr>
</thead>
<tbody>
{data.allFiles.edges.map(({ node }, index) => (
<tr key={index}>
<td>{node.relativePath}</td>
<td>{node.prettySize}</td>
<td>{node.extension}</td>
<td>{node.birthTime}</td>
</tr>
))}
</tbody>
</table>
</Layout>
);
};
export const query = graphql`
query {
allFile {
edges {
node {
relativePath
prettySize
extension
birthTime(fromNow: true)
}
}
}
}
`;
export default AllCodePage;
src/pages/all-code.js
jsx
import { graphql } from 'gatsby';
import * as React from 'react';
import { Layout } from '../components/layout';
const AllCodePage = ({ data }) => {
return (
<Layout>
<table>
<thead>
<tr>
<th>relativePath</th>
<th>prettySize</th>
<th>extension</th>
<th>birthTime</th>
</tr>
</thead>
<tbody>
{data.allFiles.edges.map(({ node }, index) => (
<tr key={index}>
<td>{node.relativePath}</td>
<td>{node.prettySize}</td>
<td>{node.extension}</td>
<td>{node.birthTime}</td>
</tr>
))}
</tbody>
</table>
</Layout>
);
};
export const query = graphql`
query {
allFile {
edges {
node {
relativePath
prettySize
extension
birthTime(fromNow: true)
}
}
}
}
`;
export default AllCodePage;